Page 1 of 1
Drawing lines and link them for moving and rotating
Posted: Thu Mar 24, 2011 4:20 pm
by 16657923
Hi,
1)
I would like to draw lines on teechart and with a command, duplicate selected line and link it to parent. So after that when I move the first line, the second is moved too (like with horizontal or vertical parallel style)
How can I do that ?
In the code bellow I write the duplicate line function but lines are not linked between them so when I move the first one, the second one does not move
Code: Select all
Private Sub Command1_Click()
Dim currentLineIndex As Integer
TChart1.Tools.Items(0).asDrawLine.SelectNewLines = True
currentLineIndex = TChart1.Tools.Items(0).asDrawLine.Selected
Dim oLine As IDrawLine
Set oLine = TChart1.Tools.Items(0).asDrawLine.Lines.Items(currentLineIndex)
With oLine
Call TChart1.Tools.Items(0).asDrawLine.AddLine(.StartPos.X, .StartPos.Y - 100, .EndPos.X, .EndPos.Y - 100)
End With
Dim nLine As IDrawLine
Set nLine = TChart1.Tools.Items(0).asDrawLine.Lines.Items(currentLineIndex + 1)
With nLine
.Pen.Color = oLine.Pen.Color
.Pen.Style = oLine.Pen.Style
End With
End Sub
2)
I would like to rotate selected lines with mouse. How can I do that ?
Thanks a lot for your help
Kind regards,
Guilz
ps : I use Teechart v8
(because v2010 is not very stable actually
)
Re: Drawing lines and link them for moving and rotating
Posted: Mon Mar 28, 2011 11:23 am
by yeray
Hello Guilz,
Using the v2010, the code bellow drags the drawn lines correctly.
Could you please check it for you?
Code: Select all
Dim oLine, nLine As IDrawLine
Private Sub Form_Load()
TChart1.Aspect.View3D = False
TChart1.AddSeries scLine
TChart1.Series(0).FillSampleValues
TChart1.Tools.Add tcDrawLine
End Sub
Private Sub TChart1_OnDrawLineToolLineDrag()
Dim currentLineIndex As Integer
currentLineIndex = TChart1.Tools.Items(0).asDrawLine.Selected
If currentLineIndex > -1 Then
Set oLine = TChart1.Tools.Items(0).asDrawLine.Lines.Items(currentLineIndex)
If currentLineIndex Mod 2 Then
Set nLine = TChart1.Tools.Items(0).asDrawLine.Lines.Items(currentLineIndex - 1)
nLine.StartPos.Y = oLine.StartPos.Y + 100
nLine.EndPos.Y = oLine.EndPos.Y + 100
Else
Set nLine = TChart1.Tools.Items(0).asDrawLine.Lines.Items(currentLineIndex + 1)
nLine.StartPos.Y = oLine.StartPos.Y - 100
nLine.EndPos.Y = oLine.EndPos.Y - 100
End If
nLine.StartPos.X = oLine.StartPos.X
nLine.EndPos.X = oLine.EndPos.X
TChart1.Repaint
End If
End Sub
Private Sub TChart1_OnDrawLineToolNewLine()
Dim currentLineIndex As Integer
TChart1.Tools.Items(0).asDrawLine.SelectNewLines = True
currentLineIndex = TChart1.Tools.Items(0).asDrawLine.Selected
Set oLine = TChart1.Tools.Items(0).asDrawLine.Lines.Items(currentLineIndex)
With oLine
TChart1.Tools.Items(0).asDrawLine.AddLine .StartPos.X, .StartPos.Y - 100, .EndPos.X, .EndPos.Y - 100
End With
Set nLine = TChart1.Tools.Items(0).asDrawLine.Lines.Items(currentLineIndex + 1)
With nLine
.Pen.Color = oLine.Pen.Color
.Pen.Style = oLine.Pen.Style
End With
oLine.Pen.Style = psNothing
oLine.Pen.Style = psSolid
End Sub
If it works fine, you should do something similar to implement a rotation.
Re: Drawing lines and link them for moving and rotating
Posted: Mon Mar 28, 2011 12:20 pm
by 16657923
Hello Yeray,
Thanks for your answer, it's working pretty well
I have an issue now with the Axis Scroll tools with your code: when I try to move the bottom axis, a new line is drawn !
So I can't move the chart with the Axis Scroll tools...
How can I fix it ?
King Regards,
Guilz
Re: Drawing lines and link them for moving and rotating
Posted: Mon Mar 28, 2011 1:02 pm
by 16657923
The 2nd thing is I would like to move only 1 line when I press ctrl button.
How can I do that Yeray ?
Thank you
Guilz
Re: Drawing lines and link them for moving and rotating
Posted: Wed Mar 30, 2011 7:52 am
by yeray
Hello Guilz,
The easiest would probably be to use the Key events to activate/deactivate the EnableDraw and EnableSelect properties:
Code: Select all
Dim oLine, nLine As IDrawLine
Private Sub Form_Load()
TChart1.Aspect.View3D = False
TChart1.AddSeries scLine
TChart1.Series(0).FillSampleValues
TChart1.Tools.Add tcDrawLine
TChart1.Tools.Items(0).asDrawLine.EnableDraw = False
TChart1.Tools.Items(0).asDrawLine.EnableSelect = False
End Sub
Private Sub TChart1_OnDrawLineToolLineDrag()
Dim currentLineIndex As Integer
currentLineIndex = TChart1.Tools.Items(0).asDrawLine.Selected
If currentLineIndex > -1 Then
Set oLine = TChart1.Tools.Items(0).asDrawLine.Lines.Items(currentLineIndex)
If currentLineIndex Mod 2 Then
Set nLine = TChart1.Tools.Items(0).asDrawLine.Lines.Items(currentLineIndex - 1)
nLine.StartPos.Y = oLine.StartPos.Y + 100
nLine.EndPos.Y = oLine.EndPos.Y + 100
Else
Set nLine = TChart1.Tools.Items(0).asDrawLine.Lines.Items(currentLineIndex + 1)
nLine.StartPos.Y = oLine.StartPos.Y - 100
nLine.EndPos.Y = oLine.EndPos.Y - 100
End If
nLine.StartPos.X = oLine.StartPos.X
nLine.EndPos.X = oLine.EndPos.X
TChart1.Repaint
End If
End Sub
Private Sub TChart1_OnDrawLineToolNewLine()
Dim currentLineIndex As Integer
TChart1.Tools.Items(0).asDrawLine.SelectNewLines = True
currentLineIndex = TChart1.Tools.Items(0).asDrawLine.Selected
Set oLine = TChart1.Tools.Items(0).asDrawLine.Lines.Items(currentLineIndex)
With oLine
TChart1.Tools.Items(0).asDrawLine.AddLine .StartPos.X, .StartPos.Y - 100, .EndPos.X, .EndPos.Y - 100
End With
Set nLine = TChart1.Tools.Items(0).asDrawLine.Lines.Items(currentLineIndex + 1)
With nLine
.Pen.Color = oLine.Pen.Color
.Pen.Style = oLine.Pen.Style
End With
oLine.Pen.Style = psNothing
oLine.Pen.Style = psSolid
End Sub
Private Sub TChart1_OnKeyDown(ByVal KeyCode As Long, ByVal Shift As TeeChart.EShiftState)
If KeyCode = KeyCodeConstants.vbKeyShift Then
TChart1.Tools.Items(0).asDrawLine.EnableDraw = True
TChart1.Cursor = -3
End If
If KeyCode = KeyCodeConstants.vbKeyControl Then
TChart1.Tools.Items(0).asDrawLine.EnableSelect = True
End If
End Sub
Private Sub TChart1_OnKeyUp(ByVal KeyCode As Long, ByVal Shift As TeeChart.EShiftState)
If KeyCode = KeyCodeConstants.vbKeyShift Then
TChart1.Tools.Items(0).asDrawLine.EnableDraw = False
TChart1.Cursor = -2
End If
If KeyCode = KeyCodeConstants.vbKeyControl Then
TChart1.Tools.Items(0).asDrawLine.EnableSelect = False
End If
End Sub
Re: Drawing lines and link them for moving and rotating
Posted: Thu Mar 31, 2011 12:53 pm
by 16657923
Hi Yeray,
It's not good for me but it's probably beacause i'm not clear
My need: be able to select and move only 1 line
when ctrl key is down only. In your code when i move 1 line the second one is moved too.
This need is to change the distance between 2 lines pressing ctrl key and moving 1 line...
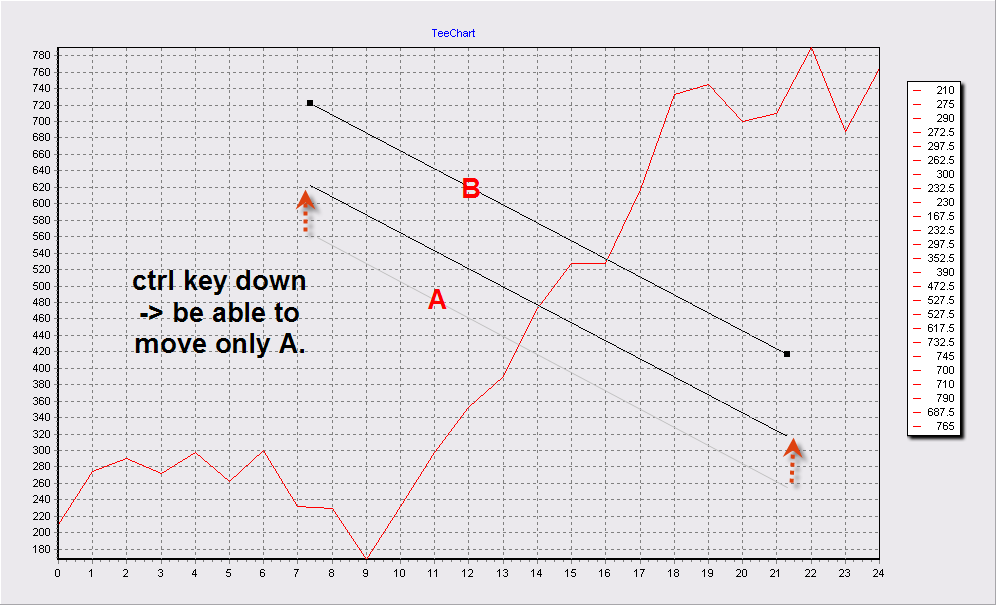
- line.png (36.55 KiB) Viewed 16319 times
Kind Regards Yeray
Guilz
Re: Drawing lines and link them for moving and rotating
Posted: Fri Apr 01, 2011 8:01 am
by yeray
Hi Guilz,
Ok, Ctrl key to move up/down each line individually. Playing a little bit with the last code it could be possible:
Code: Select all
Dim oLine, nLine As IDrawLine
Private Sub Form_Load()
TChart1.Aspect.View3D = False
TChart1.AddSeries scLine
TChart1.Series(0).FillSampleValues
TChart1.Tools.Add tcDrawLine
TChart1.Tools.Items(0).asDrawLine.EnableDraw = False
TChart1.Tools.Items(0).asDrawLine.EnableSelect = False
End Sub
Private Sub TChart1_OnDrawLineToolLineDrag()
ForceLine
End Sub
Private Sub TChart1_OnDrawLineToolNewLine()
Dim currentLineIndex As Integer
TChart1.Tools.Items(0).asDrawLine.SelectNewLines = True
currentLineIndex = TChart1.Tools.Items(0).asDrawLine.Selected
Set oLine = TChart1.Tools.Items(0).asDrawLine.Lines.Items(currentLineIndex)
With oLine
TChart1.Tools.Items(0).asDrawLine.AddLine .StartPos.X, .StartPos.Y - 100, .EndPos.X, .EndPos.Y - 100
End With
Set nLine = TChart1.Tools.Items(0).asDrawLine.Lines.Items(currentLineIndex + 1)
With nLine
.Pen.Color = oLine.Pen.Color
.Pen.Style = oLine.Pen.Style
End With
oLine.Pen.Style = psNothing
oLine.Pen.Style = psSolid
End Sub
Private Sub TChart1_OnKeyDown(ByVal KeyCode As Long, ByVal Shift As TeeChart.EShiftState)
If KeyCode = KeyCodeConstants.vbKeyShift Then
TChart1.Tools.Items(0).asDrawLine.EnableDraw = True
TChart1.Cursor = -3
End If
If KeyCode = KeyCodeConstants.vbKeyControl Then
TChart1.Tools.Items(0).asDrawLine.EnableSelect = True
End If
End Sub
Private Sub TChart1_OnKeyUp(ByVal KeyCode As Long, ByVal Shift As TeeChart.EShiftState)
If KeyCode = KeyCodeConstants.vbKeyShift Then
TChart1.Tools.Items(0).asDrawLine.EnableDraw = False
TChart1.Cursor = -2
End If
If KeyCode = KeyCodeConstants.vbKeyControl Then
TChart1.Tools.Items(0).asDrawLine.EnableSelect = False
End If
End Sub
Private Sub TChart1_OnMouseMove(ByVal Shift As TeeChart.EShiftState, ByVal X As Long, ByVal Y As Long)
ForceLine
End Sub
Private Sub ForceLine()
Dim currentLineIndex As Integer
currentLineIndex = TChart1.Tools.Items(0).asDrawLine.Selected
If currentLineIndex > -1 Then
Set oLine = TChart1.Tools.Items(0).asDrawLine.Lines.Items(currentLineIndex)
If currentLineIndex Mod 2 Then
Set nLine = TChart1.Tools.Items(0).asDrawLine.Lines.Items(currentLineIndex - 1)
Else
Set nLine = TChart1.Tools.Items(0).asDrawLine.Lines.Items(currentLineIndex + 1)
End If
oLine.StartPos.X = nLine.StartPos.X
oLine.EndPos.X = nLine.EndPos.X
TChart1.Repaint
End If
End Sub
The result isn't perfect. The dragged line is shown in it's temporal location and redrawn in its forced final location giving a flickering result.
I don't see how to avoid this easily. However, an option would probably be to hide the dragged line and use custom drawing techniques to draw the final location while dragging.
Re: Drawing lines and link them for moving and rotating
Posted: Fri Apr 01, 2011 8:27 am
by 16657923
Hi Yeray,
Thank you to try to find a solution - I can't use your code because it is not working well for me (flickering, can't move the 2 lines at the same time when ctrl key is not pressed...)
Kind regards,
Guilz
Re: Drawing lines and link them for moving and rotating
Posted: Fri Apr 01, 2011 11:27 am
by narcis
Hi Guilz,
I'm taking up on this and I may be missing something but what about doing something like this? When selecting a line you can either drag or modify it. My suggestion is simpler than what was discussed here. If it doesn't fit your needs please let me know what's missing.
Code: Select all
Private Sub Form_Load()
TChart1.Aspect.View3D = False
TChart1.AddSeries scLine
TChart1.Series(0).FillSampleValues
TChart1.Tools.Add tcDrawLine
TChart1.Tools.Items(0).asDrawLine.EnableSelect = False
End Sub
Private Sub TChart1_OnKeyDown(ByVal KeyCode As Long, ByVal Shift As TeeChart.EShiftState)
TChart1.Tools.Items(0).asDrawLine.EnableSelect = (Shift = ssCtrl)
End Sub
Private Sub TChart1_OnKeyUp(ByVal KeyCode As Long, ByVal Shift As TeeChart.EShiftState)
TChart1.Tools.Items(0).asDrawLine.EnableSelect = False
End Sub
Thanks in advance.
Re: Drawing lines and link them for moving and rotating
Posted: Fri Apr 01, 2011 11:40 am
by 16657923
Hi Narcis,
Thanks for your contribution but It does not respond to my need (be able, when I draw 1 line to draw a second line automaticaly and after, when I move 1 line the second line is moved too and third, when I press ctrl key and clic on 1 line, only this line is moved (to change the distance between line A and B)
Please try the code I made, it's working pretty well now...Perhaps we can optimize it...
Guilz
Code: Select all
Dim oLine As IDrawLine
Dim nLine As IDrawLine
Dim CTRL_KEY_DOWN As Boolean
Dim dicHeight As New Scripting.Dictionary
Const DEFAULT_HEIGHT As Long = 100
Private Sub Form_Load()
TChart1.Aspect.View3D = False
TChart1.AddSeries scLine
TChart1.Series(0).FillSampleValues
TChart1.Tools.Add tcDrawLine
End Sub
Private Sub TChart1_OnDrawLineToolLineDrag()
Dim currentLineIndex As Integer
currentLineIndex = TChart1.Tools.Items(1).asDrawLine.Selected
If currentLineIndex > -1 Then
Set oLine = TChart1.Tools.Items(1).asDrawLine.Lines.Items(currentLineIndex)
If CTRL_KEY_DOWN = True Then
Debug.Print ""
' Deplacement d'1 seule ligne
Else
Dim H As Long
If dicHeight.Exists(currentLineIndex) Then
H = dicHeight.Item(currentLineIndex)
Else
H = DEFAULT_HEIGHT
End If
' Nouvelle ligne
If currentLineIndex Mod 2 Then
Set nLine = TChart1.Tools.Items(1).asDrawLine.Lines.Items(currentLineIndex - 1)
nLine.StartPos.Y = oLine.StartPos.Y + H
nLine.EndPos.Y = oLine.EndPos.Y + H
Else
Set nLine = TChart1.Tools.Items(1).asDrawLine.Lines.Items(currentLineIndex + 1)
nLine.StartPos.Y = oLine.StartPos.Y - H
nLine.EndPos.Y = oLine.EndPos.Y - H
End If
nLine.StartPos.X = oLine.StartPos.X
nLine.EndPos.X = oLine.EndPos.X
TChart1.Repaint
End If
End If
End Sub
Private Sub TChart1_OnDrawLineToolNewLine()
Dim currentLineIndex As Integer
Dim H As Long
TChart1.Tools.Items(1).asDrawLine.SelectNewLines = True
currentLineIndex = TChart1.Tools.Items(1).asDrawLine.Selected
Set oLine = TChart1.Tools.Items(1).asDrawLine.Lines.Items(currentLineIndex)
With oLine
H = DEFAULT_HEIGHT
TChart1.Tools.Items(1).asDrawLine.AddLine .StartPos.X, .StartPos.Y - H, .EndPos.X, .EndPos.Y - H
If dicHeight.Exists(currentLineIndex) = False Then
dicHeight.Add currentLineIndex, H
dicHeight.Add currentLineIndex + 1, -H
End If
End With
Set nLine = TChart1.Tools.Items(1).asDrawLine.Lines.Items(currentLineIndex + 1)
With nLine
.Pen.Color = oLine.Pen.Color
.Pen.Style = oLine.Pen.Style
End With
oLine.Pen.Style = psNothing
oLine.Pen.Style = psSolid
End Sub
Private Sub TChart1_OnKeyDown(ByVal KeyCode As Long, ByVal Shift As TeeChart.EShiftState)
Debug.Print "down " & KeyCode, Shift
If KeyCode = 17 And Shift = 4 Then CTRL_KEY_DOWN = True
End Sub
Private Sub TChart1_OnKeyUp(ByVal KeyCode As Long, ByVal Shift As TeeChart.EShiftState)
Debug.Print "up " & KeyCode, Shift
If KeyCode = 17 And Shift = 0 Then
Dim currentLineIndex As Integer
currentLineIndex = TChart1.Tools.Items(1).asDrawLine.Selected
If currentLineIndex > -1 Then
Set oLine = TChart1.Tools.Items(1).asDrawLine.Lines.Items(currentLineIndex)
Dim H As Long
If currentLineIndex Mod 2 Then
Set nLine = TChart1.Tools.Items(1).asDrawLine.Lines.Items(currentLineIndex - 1)
H = nLine.EndPos.Y - oLine.EndPos.Y
If dicHeight.Exists(currentLineIndex) Then
dicHeight.Remove (currentLineIndex)
dicHeight.Remove (currentLineIndex - 1)
dicHeight.Add currentLineIndex, H
dicHeight.Add currentLineIndex - 1, -H
End If
Else
Set nLine = TChart1.Tools.Items(1).asDrawLine.Lines.Items(currentLineIndex + 1)
H = oLine.EndPos.Y - nLine.EndPos.Y
If dicHeight.Exists(currentLineIndex) Then
dicHeight.Remove (currentLineIndex)
dicHeight.Remove (currentLineIndex + 1)
dicHeight.Add currentLineIndex, H
dicHeight.Add currentLineIndex + 1, -H
End If
End If
End If
CTRL_KEY_DOWN = False
End If
End Sub
Re: Drawing lines and link them for moving and rotating
Posted: Mon Apr 04, 2011 10:43 am
by yeray
Hello Guilz,
So this is working as you would like, isn't it? If not, please, try to explain us what aspect would you exactly want to optimize.